2020.코딩일지
[JS/Node] callback/Promise/async,await [BEB 6th] 본문
728x90
[BEB 6th]000일차
코드스테이츠 블록체인 부트캠프 6기
👌Async비동기가 좋은 것은 알겠는데.. 순서를 제어하고 싶은 경우엔? ... 🫶최종결론은 `async,await`
비동기예시
더보기
// 비동기씨는 효율적이긴한데, 언제끝날지 예측이 불가하다
const printString = (string) => {
setTimeout(() => {
console.log(string);
}, Math.floor(Math.random() * 100) + 1);
};
const printAll = () => {
printString("A");
printString("B");
printString("C");
};
printAll();
콜백예시
더보기
// -----------비동기는 효율적이나, 언제끝날지 예측이 불가하다.그래서 콜백이 필요함
// -----------그래서 순서제어때문에 콜백을 썼더니 콜백지옥주의...
const printString = (string, callback) => {
setTimeout(() => {
console.log(string);
callback()
}, Math.floor(Math.random() * 100) + 1);
};
const printAll = () => {
printString("A", () => {
printString("B", () => {
printString("C", () => {})
})
})
}
printAll();
Promise예시
더보기
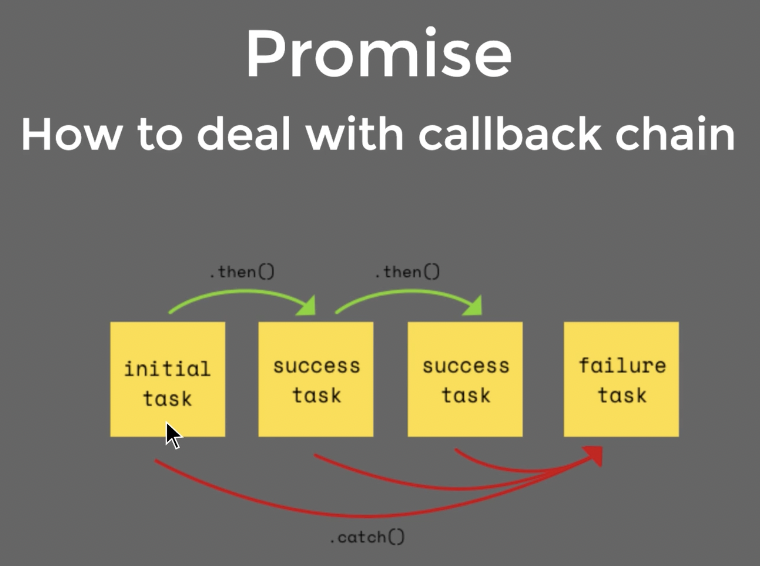
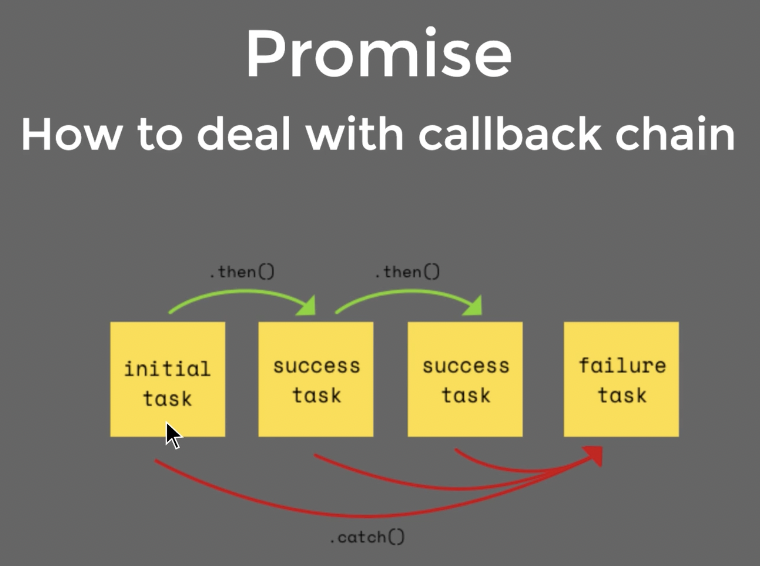
// -----------비동기는 언제끝날지 예측이 불가하다.그래서 콜백이 필요함
// -----------그래서 순서제어때문에 콜백을 썼더니 콜백지옥주의...
// -----------콜백지옥에서 벗어나려고 "promise"(resolve,reject:try-catch) '.then.catch.finally'
const printString = (string) => {
return new Promise((resolve, reject) => {
//reject에러가 있을경우엔, try-catch문으로 잡아주기.
setTimeout(() => {
console.log(string);
resolve();
}, Math.floor(Math.random() * 100) + 1);
});
};
//이것이 promise chaining
const printAll = () => {
printString("A")
.then(() => {
return printString("B");
})
.then(() => {
return printString("C");
});
}; //마지막 .catch로 모든에러를 한번에 잡을 수 있다!!
//return처리를 안하면 또 promiseHell주의 ㅋㅋㅋ
printAll();
async와 await 예시
더보기
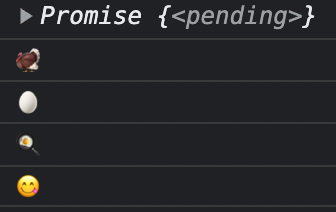
// -----------비동기는 언제끝날지 예측이 불가하다.그래서 콜백이 필요함
// -----------그래서 순서제어때문에 콜백을 썼더니 콜백지옥주의...
// -----------콜백지옥에서 벗어나려고 "promise" '.then.catch.finally'
//promise와 비동기적으로 돌아가는데 표현을 동기적으로 할 수 있어서 "async와 await"를 쓴다
function gotoFarm() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("🦃");
}, 500);
});
}
function getEgg() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("🥚");
}, 400);
});
}
function eatLunch() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("🍳");
}, 300);
});
}
function ImHappy() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("😋");
}, 500);
});
}
const result = async () => {
const one = await gotoFarm();
console.log(one);
const two = await getEgg();
console.log(two);
const three = await eatLunch();
console.log(three);
const four = await ImHappy();
console.log(four);
};
result();
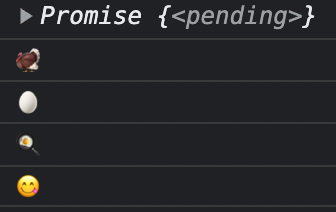
콜백이란? 다른 함수A의 전달인자(argument)로 넘겨주는 함수B
//콜백이란? 다른 함수A의 전달인자(argument)로 넘겨주는 함수B
function B() {
console.log('나왔어!');
}
function A(callback) {
callback();
}
A(B); //나왔어!
//callback in action1: 반복 실행하는 함수 (iterator)
[1,2,3].map((el, index) => el * el); //1,4,9
//callback in action2: DOM에서 이벤트에 따른 함수(event handler)
document.querySelector('#btn').addEventListener('click', function(e){
console.log('버튼이클릭되었습니다.');
});
// ----callback error handling Design
const somethingGonnaHappen = (callback) => {
waitngUntilSomethingHappens();
if (isSomthingGood) {
callback(null, something); //보통 에러를 앞에, 데이터결과값
}
if (isSomethinBad) {
callback(something, null); //에러났을경우 결과값을 에러로표시, 데이터결과값은null
}
};
somethingGonnaHappen((err, data) => {
if (err) {
console.log("ERR!!");
return;
}
return data;
});
'JS & React' 카테고리의 다른 글
[React]코딩앙마-voca-(Read - GET)useEffect(),API연결 (0) | 2022.07.30 |
---|---|
[JS/Node]PART2-Node.js의fs모듈(callback,promise,async) [BEB 6th] (0) | 2022.07.26 |
[JS/Node] 비동기 [BEB 6th] (0) | 2022.07.25 |
[!!js]자바스크립트JavaScript 느낌표두개 연산자Operator (0) | 2022.07.24 |
[에러해결]Module not found: Error: Can't resolve './reportWebVitals' (0) | 2022.07.23 |
Comments