2020.코딩일지
[JS/Node] 비동기 [BEB 6th] 본문
코드스테이츠 블록체인 부트캠프 6기
동기적(synchronous) 뒷사람의 시작시점과 앞사람의 완료시점이 같은 상황. blocking발생
//동기적인 카페에서의 상황😡
function waitSync(ms) {
var start = Date.now();
var now = start;
while (now - start < ms) {
now = Date.now();
}
}// 현재시각과 시작시각을 비교하여 ms범위 내에서 무한루프를 도는 blocking함수
function drink(person, coffee) {
console.log(person + "는 " + coffee + "를 마십니다");
}
function orderCoffeeSync(coffee) {
console.log(coffee + "가 접수되었습니다");
waitSync(2000);
return coffee;
}
let customers = [
{
name: "Anne",
request: "쑥떡프라푸치노",
},
{
name: "Json",
request: "아메리카노",
},
];
//call synchronously
customers.forEach(function (customer) {
let coffee = orderCoffeeSync(customer.request);
drink(customer.name, coffee);
});
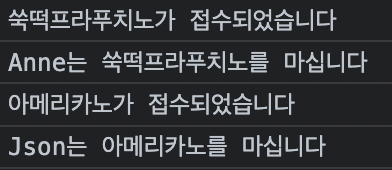
비동기적(Asynchronous execution) JS장점:non-blocking하이브리드!열심히일한다. 단점:종료시점뒤죽박죽
<비동기사례> 🌻네트워크요청 백그라운드 실행, 로딩 창 등의 작업 / 인터넷에서 서버로 요청을 보내고, 응답을 기다리는 작업 / 큰 용량의 파일을 로딩하는 작업 DOM Element의 이벤트핸들러 (마우스,키보드입력 click, keydown) 타이머API (setTimeout), 애니메이션API(requestAnimationFrame) 서버자원요청 및 응답 (fetchAPI, AJAX(XHR)) |
//비동기적인 카페!🥤 한번에 주문받고, 한번에 메뉴나옴 ㅋ
function waitAsync(callback, ms) {
setTimeout(callback, ms);
} //특정시간 이후에 callback함수가 실행되게끔 하는 브라우저 내장 기능.
function drink(person, coffee) {
console.log(person + "는 " + coffee + "를 마십니다");
}
//💛비동기
function orderCoffeeASync(menu, callback) {
console.log(menu + "가 접수되었습니다.");
waitAsync(function () {
callback(menu);
}, 2000);
}
let customers = [
{
name: "Anne",
request: "쑥떡프라푸치노",
},
{
name: "Json",
request: "아메리카노",
},
];
//call Asynchronously + 콜백함수를 넣어봄
customers.forEach(function (customer) {
orderCoffeeASync(customer.request, function (coffee) {
drink(customer.name, coffee);
});
});
비동기 흐름은 callback, promise, async/await 중 하나의 문법을 이용하여 구현할 수 있다.
💃Achievemenrt Goals
blocking/ non-blocking
synchronous / asynchronous
callback 함수 전달 방법
method chaining(Array의 map, filter등을 연결해서 쓰는 법)
-
어떤경우에 중첩된 callback이 발생하는가?
중첩된 callback의 단점, Promise의 장점
Promise 사용 패턴을 이해하자.
- `resolve`, `reject`의 의미와 `then`, `catch`와의 관계를 이해.
- Promise에서 인자를 넘기는 방법
- Promise의 세 가지 상태 이해
- `Promise.all`의 사용법 이해
`async / await` keyword에 대해 이해하고, 작동 원리를 이해
Node.js의 `fs`모듈의 사용법 이해
🤸♀️Advanced 브라우저의 비동기 함수 작동 원리
Event Loop velog.io/@tit
Philip Roberts: Help, I'm stuck in an event-loop
//재명님의코드
//배열 작성
const arrFunc = [];
for (let i = 0; i < 5; i++) {
const func = (resolve) => {
console.log(`처리 ${i} 시작`, new Date().toLocaleTimeString());
// 0~2초 지연
const delayMsec = 2000 * Math.random();
// 지연 처리
setTimeout(() => {
console.log(`처리 ${i} 완료`, new Date().toLocaleTimeString());
resolve();
}, delayMsec);
};
// 배열 저장
arrFunc.push(func);
}
console.log(arrFunc);
// 함수를 포함한 배열을 Promise 배열로 변환
const arrPromise = arrFunc.map((func) => new Promise(func));
//😎각각의 func에 Promise를 적용하겠다
console.log(arrPromise);
// 병렬 처리 실행
Promise.all(arrPromise).then(() => {
console.log('모든 작업이 완료되었습니다.');
});
'JS & React' 카테고리의 다른 글
[JS/Node]PART2-Node.js의fs모듈(callback,promise,async) [BEB 6th] (0) | 2022.07.26 |
---|---|
[JS/Node] callback/Promise/async,await [BEB 6th] (0) | 2022.07.26 |
[!!js]자바스크립트JavaScript 느낌표두개 연산자Operator (0) | 2022.07.24 |
[에러해결]Module not found: Error: Can't resolve './reportWebVitals' (0) | 2022.07.23 |
React실습 - react-twittler-state-props (0) | 2022.07.17 |